Python Tutorial: Learn Python Programming language
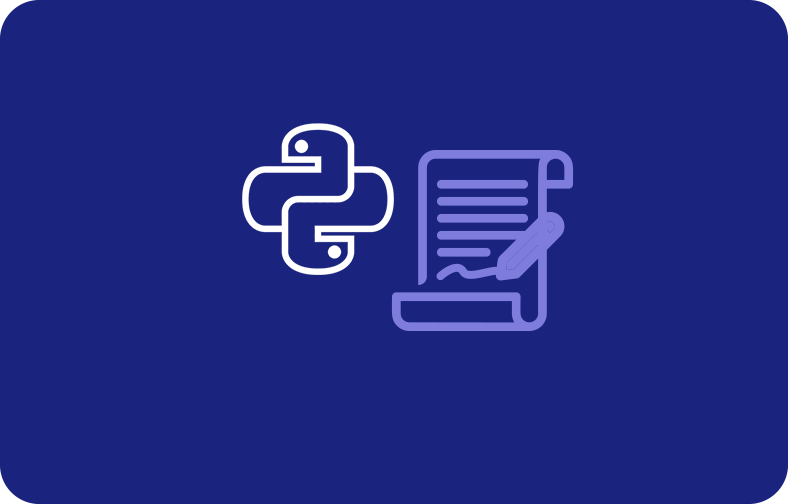
Python programming has become part and parcel of the current technology arena, transforming how companies handle automation, data analysis, and software development. Python is a powerful general-purpose technology that is very flexible and can support businesses in most industries facing the digital era.
Python is known for its readability and understanding; it's useful for developers with any level of experience. Its simple and powerful syntax makes It effective, which is good for cutting costs and development time. Due to its simplicity and a wide range of libraries and frameworks, Python has been the most commonly used programming language for many applications.
-
- Python Origin: A Brief History
- Getting Started With Python Programming Language
- Python Data Types
- Writing your first Python Program to Learn the Python Programming
- Use of Python Programming in Different Fields
- Python as Compared to Other Programming Languages
- Best Way to Learn Python
- Python and VPSServer
One significant advantage of Python is its ability to manage challenging data analysis and manipulation jobs efficiently. Libraries like Pandas and Numpy in Python help businesses gain insightful knowledge from large datasets, facilitating well-informed decision-making and strategic planning.
Furthermore, Python is a functional programming language for developing perceptive data visualizations that appeal to stakeholders due to its interaction with potent visualization tools like Matplotlib and Seaborn.
Python is a powerful tool for web development and data research. Frameworks like Django and Flask help businesses quickly and effectively develop reliable web apps and APIs. Python's asynchronous programming features and flexibility allow for smooth scaling and performance improvement for web-based solutions.
Python is more than just a programming language; it's a digital landscape catalyst that spurs innovation and transformation, enabling businesses to prosper in a rapidly changing technological environment.
Python Origin: A Brief History
Python was developed in the late 1980s when a Dutch programmer, Guido van Rossum, had the idea to create a suitable programming language that would particularly focus on readability, flexibility, and simplicity.
The first version, Python 0.9.0, appeared in February 1991 and already included several major features of the Python language: modules, functions, and exceptions. "The Zen of Python" explains the philosophy of Python and says that programming should be simple, clear, and convenient to use.
Python has a checkered development history that has spread over several years. More recent big releases are Python 2.0, released in 2000; Python 2.0, which added list comprehensions and a garbage collection system for collecting reference cycles; and Python 3.0, released in 2008. Python 3.0 aimed to eliminate redundancy and inconsistency inside the language, introducing big changes that were not backward compatible.
The combination of all these factors significant standard libraries, vibrant community, and easy learning curve—worked to bring massive popularity to Python in the 2000s. That made it the all-time favorite programming language for automation, data science, and web development.
Python is among the most popular and widely used programming languages today. Its language structure, combined with ample libraries and an active community, contributed greatly to its development and widespread use in different industries, really setting it as one of the genuine technological giants in programming history.
Getting Started With Python Programming Language
Starting with Python opens the door to infinite possibilities and an expression of creativity. With a great range of exciting opportunities, Python welcomes professional programmers eager to uncover more of their potential and newbies who are just taking the first baby steps in coding.
Python is easy and flexible, which makes it a great starting point for newcomers and quite a powerful tool for experienced programmers. Thanks to its simple and accessible grammar, you can focus on problem-solving and creating elegant Python solutions instead of getting mired down in complex code.
Setting Up Your Environment
Installation Python is user-friendly, making it easy to start. The setup process is simple, so you can start coding immediately—whether using Anaconda, a cloud-based development environment or installing Python locally on your computer.
Exploring the Basics
Learn about the basic building pieces of Python as you go further into the language. To begin your journey into coding with a solid foundation, educate yourself on variables, data types, and basic operations. The Python REPL, an interactive shell for Python, lets you experiment in real-time and get a hands-on understanding of every line of code.
Dive Deeper Into Structure And Functions
Once you have a firm understanding of the fundamentals, develop dynamic and responsive programs by delving deeper into Python's control structures, which include loops and conditional statements. Discover how functions may help you modularize your code, encourage reuse, and improve code readability—all of which are characteristics of Python's design philosophy.
Utilize the Power of Libraries
One of Python's main strengths is the large availability of libraries and frameworks that extend the language far beyond its core. Take Flask or Django for web development, pandas for data manipulation, matplotlib for data visualization, and NumPy for numerical computing—to name a few. Each library increases your capacity to code and do many different things.
Join a Python Community to Enhance Your Abilities
Participate in cooperative projects, go to coding meetups, and join active online forums to network with other Python lovers and add to the body of knowledge. The vibrant Python community, which accepts students of all backgrounds and ability levels, is evidence of the language's inclusive and encouraging culture.
Python Data Types
The foundation of Python's adaptability and strength is its strong data type system, which serves as the building block for information representation, manipulation, and storage. Python has extensive data types, each suited to particular use cases and functionality, ranging from basic integers to intricate data structures.
Numeric Data Types: From Integers to Floats
Python's numerical data types include floats, complex numbers, and integers, offering a wide range of tools for numerical calculations. The 'int' type indicates an integer, a whole number without a decimal point. For instance, x = 5 gives the variable x the value 5.
Floats, denoted by the 'float' type, are adept at handling decimal numbers. For example, y = 3.14 gives the variable y the value 3.14. Complex numbers combine a real and an imaginary component; for example, z = 2 + 3j, where 2 is the real element, and 3j is the imaginary part. Complex numbers are represented by the 'complex' type.
String Data Type
Python strings, denoted by the 'str' type, allow textual data to be processed and altered. They are encapsulated in single ("") or double ("") quotes to provide for text handling flexibility. For instance, the expression "Joe Biden" is assigned to the variable name when name = "Joe Biden".
Concatenation ("Hello, " + "World" generates "Hello, World") and slicing (message[2:5] retrieves characters from index 2 to 4) are a couple of noticeable operations in Python string
Boolean Data Type Annotations
The 'bool' type represents boolean data types, which represent logical values of True or False. In Python applications, boolean data types are crucial for decision-making and control flow.
Logical operations are made easier by boolean operators like AND, OR, and NOT. For instance, if both requirements are satisfied, result = (x > 5) and (y < 10) evaluates to True. Boolean data types are essential in conditionals, loops, and boolean algebraic expressions.
List Data Type Annotations
In Python, lists—represented by the 'list' type—allow more than one item to be stored in a single variable. They permit heterogeneous items and are mutable and organized. For instance, a list of integers, say numbers, is as follows: [1, 2, 3, 4, 5].
Lists can be used for operations like slicing (numbers[2:4] take elements from index 2 to 3), nesting (lists within lists), and adding (numbers. append(6) adds 6 to the end). These are adaptable data structures that are employed to store sets of data.
Tuple Data Type
Like lists, tuples in Python are denoted by the 'tuple' type and are immutable, meaning their elements cannot be changed once created. They are frequently utilized for fixed data sets and indicated by parenthesis (). Coordinates = (10, 20) generates a tuple of coordinates, for instance.
Tuples support iteration, unpacking (x, y = coordinates sends values to variables x and y), indexing (coordinates[0] finds the first element), and unpacking in Python. They are more immutable and perform better than lists but are less flexible.
Dictionary Data Type
Python dictionaries, represented by the 'dict' type, make key-value mapping easier and enable effective key-based data retrieval and manipulation. They are made up of key-value pairs and are encased in curly braces {}. A dictionary is created, for instance, by person = {'name': 'Joe', 'age': 30}.
Dictionaries facilitate activities like adding or changing key-value pairs (person['email'] = 'joe@example.com' adds an email key), iterating over keys or values, and accessing values (person['name'] retrieves 'John'). They are perfect for implementing associative arrays and structuring structured data.
Writing your first Python Program to Learn the Python Programming
Writing your program is an exciting and fundamental first step in learning Python programming. This is the first step towards your journey into computational thinking and coding. You'll need an Integrated Development Environment (IDE) or text editor to begin writing and running your Python code.
Together, let's get started on writing a basic Python program. Launch your IDE or text editor, then start a new file. You will write your Python code in this file. The "Hello, World!" program is the customary opening program in any programming language.
It's easily written in this first code in Python; write print("Hello World") and click the 'Run' button. That's it; your first Python program has been written. Now we understand what this code does:
-
The built-in Python function print() is used to show output.
-
The text to be printed is represented by the string "Hello, World!" encircled in double-quotes.
Congratulations! If you've finished the above drill, you've successfully written and run your first Python program. This easy exercise prepares you for more challenging and fascinating programming adventures by teaching you the basics of Python's simple syntax, producing output, and executing code. Continue learning, honing, and developing your newly acquired Python abilities.
Use of Python Programming in Different Fields
Because of its durability and versatility, Python has risen to the top in various industries, influencing business practices and spurring innovation. Let's explore some key domains where Python excels and explain its application in each.
Web Development
Python is a driving dynamic force in web development, promoting efficiency and innovation. Its flexibility allows the scaling of web applications, providing much-needed support and reliability using frameworks like Flask and Django.
Python is a toolkit for developers with features that are so compelling. Its vast libraries and ease of managing databases make it the best in performing heavy functions and reducing backend logic.
The very dynamic nature of Python makes it very suitable for developing user interfaces while working on the front end, which is best with JavaScript frameworks such as React and Vue.js. Python is superior in web development; it is part of its flexibility and potency in the ability to bear on the digital world from rapid prototyping to enterprise-grade solutions.
Data Science And Analytics
Python is becoming dominant in data science and analytics, transforming insights and decision-making. It provides a robust ecosystem for statistical analysis, the creation of machine learning models, and the analysis of large datasets, complemented by libraries like NumPy, pandas, and sci-kit.
Data professionals prefer Python libraries for data cleaning, manipulation, and visualization as they are versatile and user-friendly. Python enables predictive analytics and actionable insights by integrating Jupyter Notebooks, TensorFlow, and PyTorch frameworks. It advances deep learning and artificial intelligence, making data exploration and collaboration more interactive.
Python has contributed a priceless effort to data science. It has provided big creativity and revolutionary ideas for very many enterprises, starting from simple exploratory data analysis up to predictive modeling.
AI and Machine Learning
Python is the artificial intelligence and machine learning programming language setting the pace with groundbreaking discoveries and solutions, creating tremendous changes. The huge Python libraries are open to academicians and developers, making it possible to build sophisticated neural networks, deep learning models, and prediction algorithms using TensorFlow, PyTorch, and sci-kit.
Thanks to Python's English-like syntax and rich ecosystem, AI and ML projects benefit from faster development, rapid prototyping, and seamless algorithm integration. While frameworks like Keras and OpenCV improve AI capabilities across computer vision, natural language processing, and reinforcement learning domains, Python modules make data preprocessing, model training, and evaluation easier.
Python's dominance in AI and ML highlights its crucial role in influencing the direction of technology and propelling innovation to new heights, from intelligent chatbots to self-driving cars.
Software and Game Development
Python significantly influences software and game development, inspiring creative solutions in application and gaming. The best game creation uses Python because frameworks like Pygame enable developers to create attractive 2D game projects easily.
Python's ease of use and readability make It an excellent choice when working on game assets, AI behavior implementation, or game logic. Its flexibility also extends into software development, enabling it to power jobs, automate software development processes, and expedite prototyping.
Thanks to libraries like Flask and Django, Python speeds up the creation of web applications and provides scalable solutions for startups and established organizations.
Python's ability to integrate with databases, APIs, and cloud services improves the software's functionality and increases performance and efficiency. Python's influence in software and game creation continues to innovate and change digital experiences, from independent games to business software.
Cybersecurity and Penetration Testing
Python is the go-to language for experts because it gives them incredible tools and lets them automate attacks. Think of it as the heart of what we do. With Python, we can do all sorts of things like messing with packets, scanning networks, and finding weaknesses. It's the engine behind important tools like Scapy, Nmap, and Metasploit. So, basically, Python is the key that unlocks a lot of doors in our field.
The Python scripts automate defense methodologies against cyber threats using security activities, data collection on threat intelligence, and analysis. Flexibility extends to incident response, cryptography, and web application security testing.
Cybersecurity professionals use Python for its simplicity and extendibility, enabling them to thoroughly harden systems, flush out vulnerabilities, and minimize threats effectively.
Python as Compared to Other Programming Languages
This special combination of the strength of Python, along with its versatility and simplicity, makes it stand out from the rest of the languages. C++, JavaScript, Ruby, and Rust are known for their specialties apart from Python, among other programming languages.
Ease of Learning and Readability
Python is simple to learn and understand since it is very precise, beginner-friendly, and fairly simple syntactically compared to other programming languages. In contrast to languages like C++ or Java, it requires fewer lines of code to accomplish an equivalent functionality measure. Therefore, it is less complex, and the code is more readable.
Versatility and Ecosystem
Python's large standard library and third-party packages serve numerous applications in automation, data research, web development, and artificial intelligence. Its flexibility, along with that of Django, Flask, NumPy, TensorFlow, and other frameworks, puts it at the forefront and makes it a favorite choice for many uses.
Performance and Scalability
Python's REPL, interpretative nature, and dynamic typing make it easy to experiment and prototype quickly. Compared with statically typed languages, it is easier for developers to prototype the design, try concepts, and iterate through more code.
Community and Support
The Python developer, contributor, and enthusiast community is full of life and enthusiasm. This community-powered environment provides developers at all stages of development with copious documentation, discussion forums, and web tools to encourage collaboration, knowledge sharing, and moving forward.
Performance and Scalability
Python remains considerably slower than C and C++; however, a number of implementation improvements, such as PyPy and Just-in-Time compilation, have greatly enhanced Python's speed. This allows a developer to fine-tune numerical computations with, for example, NumPy libraries in projects demanding heavy computational loads.
Integration and Interoperability
It has very high interoperability because Python integrates very well with most platforms, technologies, and other programming languages. In this context, Python can reach greater extents into ecosystems by developing it with Jython, which can be integrated with Java, Cython for interfacing with C/C++ libraries, or IronPython for .NET.
Best Way to Learn Python
Online Python Courses and Tutorials
Online tutorials and courses are excellent tools for studying Python at your own pace. Comprehensive Python classes spanning basic to advanced topics are available on platforms such as Coursera, edX, Udemy, and Python.org.
These courses frequently incorporate projects, quizzes, coding exercises, and video lectures to supplement classroom instruction. The official Python website is a great place to start because it offers many free tutorials, documentation, and interactive courses for novices.
Interactive Coding Platforms
Interactive coding resources like LeetCode, CodeCombat, and Codecademy offer practical learning opportunities for Python enthusiasts. These services make learning fun and interactive by providing gamified Python coding tasks, exercises, and projects.
For example, Codecademy's Python course helps students grasp Python syntax, data structures, and algorithms by providing them with real-time feedback and guidance. This helps students get a deeper comprehension of Python programming topics.
Python Books and Documentation
Python's principles and best practices are best understood from books and official documentation. "Python Crash Course" by Eric Matthes, "Automate the Boring Stuff with Python" by Al Sweigart, and "Fluent Python" by Luciano Ramalho are some of the books on introductory, practical, real-life application, and highly advanced Python.
The Python official documentation is a very important resource. It provides full explanations and tutorials for every module, function, and data type in Python, with which a Python student at any level must interact.
Project-Based Learning
Project-based learning can be quite effective for building a portfolio and applying Python knowledge to real-world situations. Begin with simple tasks such as developing a to-do list application, a calculator, or a pandas data analysis tool.
As your confidence grows, take on more challenging tasks with Python, such as creating a chatbot that uses natural language processing (NLP), creating a web scraper, or putting machine learning techniques to use for data analysis. Repositories and datasets for practicing and working together on Python projects are available on platforms like GitHub and Kaggle.
With the help of the above-mentioned Python learning resources, you may develop a solid foundation of Python, gain real-world experience, and become an expert Python programmer for various uses and fields.
Python and VPSServer
Hosting Python applications on VPS—for example, on a VPSServer—will enable you to scale flexibly and securely in the ideal hosting environment for web applications, APIs, and data processing. It supports frameworks like Django and Flask for effective deployment and management, where the needed infrastructure assures performance under varying loads.
VPSServer's customizable environments allow developers to install specific Python versions and dependencies, tailoring the server to their application's needs. With robust security features and easy scalability, VPSServer ensures Python applications remain secure and can grow with user demand. This combination creates a powerful, resilient, high-performing Python application environment.
Conclusion
Python is an adaptable, potent, and indispensable programming and technological language. Its ease of use, vast library, and friendly community make it the perfect option for novice and experienced developers. Whether studying via books, interactive courses, online courses, or project-based, Python provides a rich ecosystem for developing skills and solving practical problems.
Participating in the Python community, working together on projects, and continuing education through tutorials and documentation all help to improve Python programming skills. In the ever-changing world of code and technology, embracing Python opens up a world of potential, inventiveness, and limitless possibilities.
Frequently Asked Questions
How does the Python software foundation operate?
The Python Software Foundation is a nonprofit organization that ensures the community and development of Python move forward. It focuses on resource management, project funding, event planning, education promotion, oversight over the standard library, and diversification and inclusion through grants, sponsorships, and activities. The PSF ensures that the Python ecosystem is going to keep growing and being successful.
How does the Python software foundation operate?
The Python Software Foundation is a nonprofit organization that ensures the community and development of Python move forward. It focuses on resource management, project funding, event planning, education promotion, oversight over the standard library, and diversification and inclusion through grants, sponsorships, and activities. The PSF ensures that the Python ecosystem is going to keep growing and being successful.
Apart from Python, what other programming languages are used?
The software development business uses several other programming languages in addition to Python. Among these are web development with JavaScript and Java for enterprise applications, system programming and performance-critical applications with C/C++, Windows development with C#, iOS and Android development with Swift, web development with Ruby, server-side scripting with PHP, and Windows development with C#.
Is Python easy for beginners?
Yes, Python is very friendly to beginners due to the clear syntax, structure of easily understandable code, and abundant documentation. Python is perfect for learning programming ideas, developing basic skills, and switching to more complicated programming languages and domains precisely because it is simple.
How can I select the best Python tutorial for my type of learning?
Think about things like your preferred learning style (text-based tutorials, interactive platforms, videos), the degree of interaction (quizzes, hands-on coding exercises), and the particular subjects you are interested in (web development, data science, machine learning) while selecting the best Python tutorial. Try out a few tutorials to see which best suits your learning objectives and style.