Exploring Django: A Guide to Python Web Development
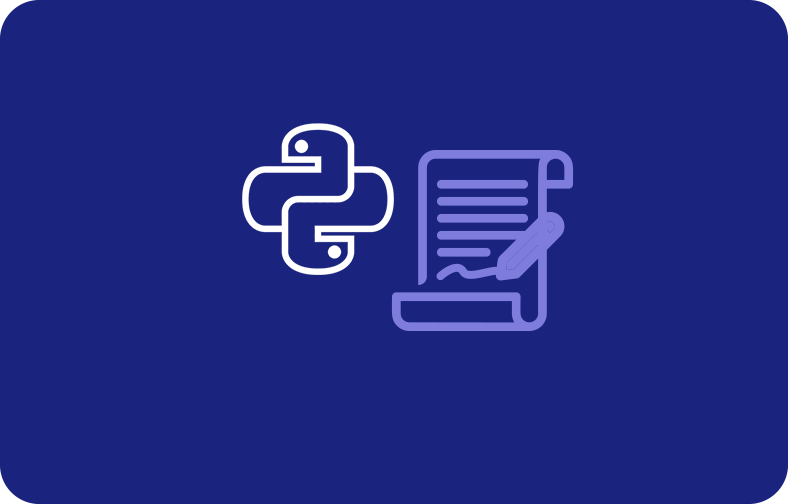
Web and software development is the design and maintenance of internet-based websites and online applications. It involves various responsibilities, including network security setting, client-side/server-side scripting, web design, and web content production. Web and software development is essential for companies and people to create an online presence, interact with users, and offer services.
Django web framework in Web Development
Django's high-level Python web framework promotes efficient development and simple, straightforward design. Created by seasoned developers, it streamlines the process of creating websites without bothersome features, freeing web developers to focus on crafting their apps rather than starting from scratch. Developers use it because it is easy to use, quick to create dependable online applications, and flexible.
The most interesting aspect of the Django concept, batteries included, is that the system already incorporates numerous functionalities that ultimately become crucial for work: an admin interface, authentication, and an ORM.
Other great things about it include safety, scalability, and very fast development time, making it the best option for projects, irrespective of their scale.
What is Django?
A high-level Python web framework called Django promotes efficient development and simple, straightforward design. Created by seasoned developers, it streamlines the process of creating websites without bothersome features, freeing developers to focus on crafting their apps rather than starting from scratch.
Developers prefer the Django web framework because it is simple, quick, and adaptable when creating reliable online applications.
Why Django for Web Development?
Benefits of Django Web Application Framework
Rapid Development
Django follows the principle of "batteries included," meaning it comes ready to ship with many features that allow for easy application set-up without the need to install and configure dozens of third-party packages. The essential features are built-in—authentication, URL routing, and ORM—to cut setup time and facilitate rapid prototyping.
Batteries Included
Django comes with many things out of the box, such as the authentication system, database servers, URL routing, template engine, etc., so the need for third-party integration is reduced, speeding the actual development.
Scalability
Django has been developed so that developers can create highly scalable web applications. The high scalability architecture has allowed Django to house high user loads and traffic volumes in projects. Major websites like Instagram and Disqus have applied Django to scale applications effectively.
Security
Django includes several development tools that are crucial in preventing unethical security implementations. It is exceptionally safe in protecting against SQL injection, clickjacking, cross-site scripting, and cross-site request forgery (CSRF). Installing this user authentication system is not too difficult because it is secure and never exposes critical info.
Versatility
Django can build a large diversity in web applications, from content management systems and social networks to scientific computing platforms and e-commerce sites. Its flexibility, with a feature set that is fully comprehensive, makes it apt for projects of different types and sizes.
Admin Interface
Django's best feature is the auto-generated admin interface, through which developers can easily manage application data with the help of the web interface. This reduces the effort of building the custom admin panel from scratch, saving a lot of time.
Community and Support
Django has a large and active community, massive documentation, third-party packages, and many developers. So, many resources can support one in continuous learning and problem-solving.
Compared with Other Web Frameworks
Django vs. Flask
Flask is a minimal, lightweight micro-framework for Python. This arms a developer with a toolkit and libraries but at the cost of burdening the developer with more configuration and setup work compared to Django, which, with a design pattern that has "batteries included," is more convenient for developers used to rather full-featured, well-thought-out frameworks—those in which everything needed to work with is also located in.
Django vs Ruby on Rails
Ruby on Rails is another web framework written in Ruby that feels somewhat the same regarding philosophy and features. The focus of the two frameworks is on rapid development, scalability, and ease of use. The fact is that Django uses Python, which is a great benefit since Python is very readable and large in almost all domains.
Django vs. Laravel
Laravel is a PHP framework with a syntax and feature set that would like to appeal to PHP developers. While equally powerful and feature-rich, Django offers the use of Python, making the Django framework extra likable to already fluent Python coders or those looking for a performance boost and a myriad of libraries that Python brings.
Django vs. Express.js
Express.js is a minimal, flexible, free, and open-source, unopinionated Node.js web application framework. It has much flexibility built in, but at the same time, it's more hands-on and less already done for you than the Django framework since Django out-of-the-box provides solutions to many common problems. It is widespread to see Django as the first pick among developers desiring a more structured and feature-rich environment.
Getting Started with Django
Installation and Setup
Python Installation
First, ensure that Python 3.6 or newer is installed on your system. The official Python website provides the Python installer and installation instructions.
You can verify that Python was successfully installed by running `python --version` in the terminal or command prompt. The version number displayed will indicate that the installation was successful.
Install Django
Once Python is installed, you can install Django. Django is installed using `pip`, the Python package installer. Open a terminal and run this command: `pip install Django'. This command will download and install the package.
To check if it's successfully installed, you can execute `django-admin --version` in the terminal; it will give you a version number of Django, hence showing that Django was already installed successfully.
Verify Installation
To verify that Django is installed and operational, run the following command in a terminal window. Open up a terminal and run: `django-admin --version`. If Django has been installed correctly, when you run the preceding command, it will display the version of the Django package that you have installed.
Basic Project Structure
When creating a new project, Django would have set up a directory structure with several pivotal files and directories for you. To be productive in working with Django, you must know this structure.
Project Directory: This is the outermost directory of the whole project. It is the main folder encompassing the project files.
manage.py: Command line utility that enables interaction with the project; start development servers, apply database migrations, or some other administrative development tasks.
Project Package Directory
It is generally the same name as your project and contains these files:
-
__init__.py: An empty file telling Python the directory is a Python package.
-
settings.py – A file that stores the configurations and settings of a project, such as the database configuration, installed applications, middleware, etc.
-
urls.py: This is where you declare the URL patterns for your project. That is, it maps the URL patterns to views.
-
wsgi.py: An entry point for the WSGI-compatible web servers to serve your project.
-
asgi.py: An entry-point for ASGI-compatible web servers to serve the project; it handles the asynchronous features of your project.
Creating Your First Django Project
Create Project
Open your terminal and run the command `django-admin startproject myproject`. Replace `myproject` with your desired project name. This command creates a new directory with the name of your project and sets up the initial project structure inside it.
Django creates a directory structure with important files and directories when you create a new project. You need to know how to work productively with Django.
Run the Development Server
Upon hitting enter, you will see that the new prompt reads: `cd my project`. Run the development server by issuing this command: `python manage.py run server`, which boots up a lightweight development server included with Django. You'll have `http://127.0.0.1:8000/` open in your browser with a Django "It worked!" page. This means the project was built, and the server is running properly.
Create an Application
A Django project will have many applications, each with a specific purpose. The command is, therefore, `python manage.py startapp myapp`. Just replace `myapp` with the name of your new application.
After doing so, the next task will be to create a new directory within the directory of your project named `myapp`. The directory will hold all the files that will prepare you to start building your application.
Setup the Application
Add your app to the `INSTALLED_APPS` setting in your project `settings.py`. Open the `settings.py` file in your project, and in the list, add the line 'myapp'. This tells Django to include your application in the project's setup.
Define Models
Django models are Python classes that define the database structure for an application.
For instance, you could have a model for a blog post with fields for the title, content, and creation date. After defining your models in `myapp/models.py`, you can create one of the `required database tables` by generating and running a migration.
Running a migration consists of two commands: `python manage.py makemigrations` for preparing the database schema changes and `python manage.py migrate`, which goes on to make those changes into the database.
Views and Templates
In Django, views are Python functions describing the application a user may interact with. Templates are HTML files that determine the structure passed from the views. For example, you can have a view in `myapp/views.py` that you would like to use to show your data.
For instance, it retrieves all the blog posts from the database and passes them to a template. Create a corresponding HTML template in the `myapp/templates/` directory to display the data.
Map URLs to Views
You can make your views available through URLs by mapping the URLs to views in `myapp/urls.py`. A URL pattern is a list of instances that define an application's URL configuration. URL instances, in turn, determine the mapping of URL patterns to views.
Add URL patterns to the project's main `urls.py` using `include`. This way, the requests for a URL belonging to your application will be dispatched to the respective views.
Suppose you follow through step by step with the given details. In that case, you will succeed in setting up Django and creating a project and an application while laying the foundation for setting models, views, and templates for building your web application.
This sets the base for further development and improvement of the developed application with more features and functionalities.
Development Ideas Using Django
Developing a Blog Application with Django
Django’s versatile toolkit makes it an excellent choice for creating a robust blog application. By leveraging Django’s ORM for data management and a templating system for dynamic content rendering, developers can define post, comment, and category models, ensuring organized data structuring.
Views can be tailored to showcase blog posts, facilitate user interactions such as commenting, and manage content presentation effectively. This admin interface provides an excellent way to easily manage most of the back-end.
Hence, productivity is high. Django's SEO-friendly features can also engage more traffic and make the blog more visible.
Creating an E-commerce Platform with Django
Django’s flexibility and security features allow developers to craft scalable e-commerce websites easily. Django’s ORM simplifies data modeling for products, orders, and user profiles, while custom views handle product listings, shopping cart functionalities, and secure checkout processes.
The payment gateway would be integrated with Stripe or PayPal to make the process seamless. Django's authentication system allows smooth user registration, login, and account management. Customizable templates and robust security measures make Django a compelling choice for building secure and user-friendly e-commerce platforms.
Django Template Language
The Django template language provides a powerful way to create dynamic and reusable HTML templates within Django web applications. It allows developers to embed Python-like code directly into HTML, facilitating the display of dynamic content such as variables, loops, conditionals, and template inheritance.
Developers can seamlessly manipulate data, format content, and control template logic with template tags and filters. Django template language promotes code readability and maintainability by separating presentation logic from business logic, enabling developers to focus on creating engaging user interfaces while ensuring efficient data rendering and manipulation.
Deploying Django app with VPSServer
Pairing Django with VPSServer's hosting solutions ensures optimal performance and scalability for your web applications. VPSServer's scalable VPS options enable you to adjust resources as needed, ensuring smooth operations during traffic spikes. With customizable server configurations and root access, developers can fine-tune settings and enhance server performance for Django projects.
What's more, VPSServer is a secure hosting platform with robust security features like firewalls and DDoS protection, operating on Django principles to keep your applications and data safe.
Additionally, an advanced global network guarantees the lowest latency and high availability for a superior user experience worldwide. Hosting with VPSServer, using the power of Django, allows developers to create and deploy efficient, reliable, and high-performance web applications in a secure hosting environment.
Conclusion
In summary, Django is a complete and powerful web development framework with many tools and features for developers to develop highly scalable, secure, and dynamic applications.
Its compliance with the DRY principle, strong ORM, built-in admin interface, and varied packages in the ecosystem make it a preference for developers seeking to cut down on development time and deliver effective web solutions.
Whether for creating complex web applications, APIs, or content management systems, Django, along with a very supportive community and excellent documentation, is a versatile framework that enables developers to put their ideas to life effectively and efficiently.
Frequently Asked Questions
Is Django suitable for web developers starting their journey?
Django can indeed be very beginner-friendly due to its high level of documentation, a pre-built-in admin interface, and the "batteries included" philosophy, which lowers the need for any external library. However, some basic knowledge of Python is suggested for a smoother learning curve.
What are the key components of Django projects?
Django projects typically consist of the project directory, manage.py utility, settings file for configurations, URL routing, views for handling requests, templates for user interface design, and models for the code organization defining data structures.
Can Django be applied in the construction of APIs?
Yes, Django can be used to write RESTful APIs. An extension, the Django REST Framework, helps with this. It allows building APIs via serializers, views, authentication, and tools.
What deployment options are available for Django applications?
Django applications can be deployed on various platforms, such as traditional web servers (like Apache or Nginx), cloud platforms (like AWS or Heroku), or specialized Django hosting providers (like VPSServer). Deployment methods often include using WSGI servers like Gunicorn or ASGI servers for asynchronous features.
How does the Django Software Foundation operate?
The Django Software Foundation is a non-profit organization that steers development with all the logistics: resources, sprint funding, events, governance, and decision-making procedures. The DSF also helps build a community through initiatives involving community engagement, diversity, and education opportunities.