How to Compare Strings in C++?
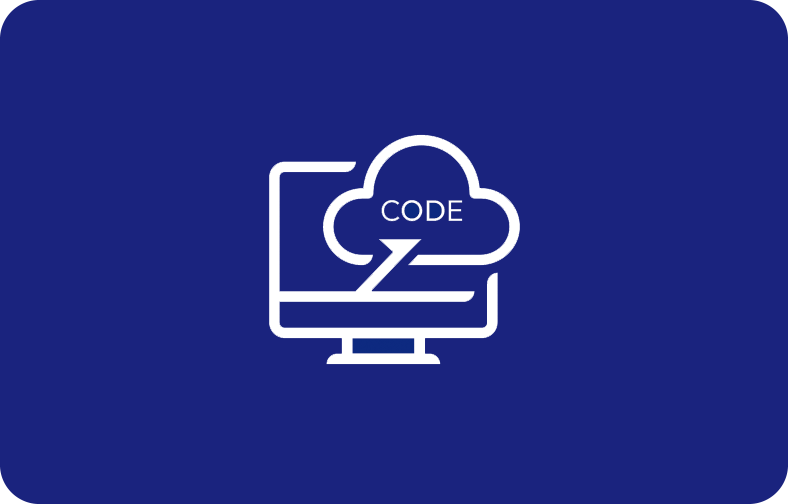
In the world of programming, different types of data are handled by programming languages. Among these, 'strings' hold significant importance across all programming languages. Strings are a type of data that's extensively used in coding. When programs are compiled, there are numerous instances where string comparison is required.
Comparing strings is a common principle, and we use it widely. Yet, various programming languages offer different methods and techniques to compare strings.
This guide will explain three methods for string comparison in C++. Let's dive in!
3 Ways to Compare Strings in C++
String comparison in C++ will be visualized by using the following methods.
-
Utilizing the 'strcmp()' function created for string comparisons.
-
The built-in 'compare()' function is designed explicitly to compare strings.
-
The third one is relational operators in C++ like '==' and '!='.
1. Using the function strcmp() in C++
In C++, there exist specific functions designed for compare two strings, among which is the function strcmp() sourced from the C library. This function plays a crucial role in comparing two strings in a lexicographical order. Lexicographical means comparing strings following character by character depending on their ASCII value. It also follows the dictionary order.
Syntax of strcmp():
strcmp() function takes input parameters as char arrays, representing C-style strings. This process is sensitive to letter case, meaning the function compares both the first and second strings.
This function return 0, negative or positive values.
int strcmp(const char *str1, const char *str2);
Return values of strcmp() function:
This function returns:
-
0 when both strings are identical.
-
< 0 (negative) when the string 1 is lexicographically less than the string 2.
-
> 0 (positive) if the string 1 is lexicographically greater than the string 2.
Example-1:
In the upcoming C++ code, start by including the header file at the beginning, such as '<cstring>'. Then, define the char array individually and assign a string to each. Additionally, in C++, the asterisk '*' accompanying array names signifies a pointer.
#include<iostream>
using namespace std;
#include<cstring>
int main() {
const char *str1 = "Apple";
const char *str2 = "Orange";
cout << "String 1: ";
cout << str1 << endl;
cout << "String 2: ";
cout << str2 << "\n";
if(strcmp(str1,str2)==0){
cout << "\nBoth strings are equal." << endl;
} else
cout << "\nThe strings are not equal." << endl;
}
Output:
Hence, the expression strcmp(str1, str2) compare the strings and results in a non-zero value because the input were two different strings, so the results are as follows:
String 1: Apple
String 2: Orange
The strings are not equal.
Example-2:
In this snippet of code, the initial and secondary strings are pre defined, just as they were previously set.
#include<iostream>
#include<cstring>
using namespace std;
int main() {
const char *str1 = "Hello";
const char *str2 = "Hello";
cout << "String 1: ";
cout << str1 << endl;
cout << "String 2: ";
cout << str2 << "\n";
if(strcmp(str1,str2)==0)
printf("\nBoth strings are equal.");
else
printf("\nThe strings are not equal.");
}
Output:
In the above code both groups of characters in the strings have the exact same ASCII values and the strcmp(str1, str2) function gives back 0. This zero represents that "Hello" word in both strings is same.
Additionally, if both arrays contain a null character when compared, they will also result in the same output.
String 1: Hello
String 2: Hello
Both strings are equal.
2. Using the compare() function in C++
C++ provides the compare() function designed specifically for comparing two strings.
Syntax of compare():
The function prototype for the compare() function is specified as follows:
int compare(const string& string_name) const;
Return values of compare():
This library function returns an integer return type:
-
0 if both strings are identical.
-
(negative value) - < 0, if the string 1 is alphabetically before the string 2 in the dictionary order.
-
(positive value) - > 0, if the string 1 is lexicographically greater than the string 1.
Example-1:
The subsequent code demonstrates the comparison of two strings utilizing a compare() function.
#include<iostream>
#include<string>
using namespace std;
int main() {
string str1("Apple");
string str2("Orange");
cout << "String 1: ";
cout << str1 << endl;
cout << "String 2: ";
cout << str2 << "\n";
int result = str1.compare(str2);
if (result == 0)
cout << "\nBoth strings are equal.";
cout << "\n";
else if (result < 0)
cout << "\nString 1 is smaller than String 2." << endl;
else
cout << "\nString 1 is greater than String 2." << endl;
}
Output:
The compare () function returns a negative value in this case because, in string comparison, string 2 is greater than string 1.
String 1: Apple
String 2: Orange
String 1 is smaller than String 2.
Example-2:
Consider the following code in which we will involve integer value inside the strings and experience the possible return values after matching cases in this situation.
#include<iostream>
#include<string>
using namespace std;
int main() {
string str1("Hello123");
string str2("Hello123");
string str3("Goodbye456");
cout << "String 1: ";
cout << str1 << "\n";
if(str1.compare(str2)==0)
cout << "\nStrings are equal." << "\n";
else
cout << "\nStrings are not equal." << endl;
cout << "String 2: ";
cout << str3 << "\n";
if(str3.compare(str1)==0)
cout << "\nStrings are equal." << "\n";
else
cout << "\nStrings are not equal." << endl;
}
Output:
Corresponding results show all the characters in this string comparison are equal, and the numeric values do not make any difference while writing in a string.
String 1: Hello123
Strings are equal.
String 2: Goodbye456
Strings are not equal.
3. Relational Operators (==, =!) in C++
In C++, relational operators like == (double equals) and != (not equals) prove invaluable for comparing strings.
Syntax of 'Double Equals' Relational Operator:
-
To verify whether two strings are equal: string1 == string2
Example-1:
Consider an example where we obtain the user input strings by utilizing a while loop.
#include<iostream>
#include<string>
using namespace std;
int main(){
string str1;
string str2;
int count=1;
while(count<3){
if(count==1){
cout << "Enter String 1: ";
cin >> str1;
} else {
cout << "Enter String 2: ";
cin >> str2;
}
count++;
}
if (str2==str1)
cout << "Strings are equal" << "\n";
else
cout << "Strings are not equal" << "\n";
}
Input/Output:
In this situation, the strings entered by the user are 'hello' and 'apple,' in that order. The '==' operator compares the two strings and returns a flag indicating that both strings are different.
Enter String 1:hello
Enter String 2:apple
Strings are not equal
Syntax of 'Not Equals' Relational Operator:
-
To check if two strings are not equal: string1 != string2
Example-2:
In this scenario, use a not equals operator '!=' and take the input string from the user. The user should give a negative number as their input.
#include<iostream>
#include<string>
using namespace std;
int main(){
string str1;
string str2;
cout << "Enter String 1: ";
cin >> str1;
cout << "Enter String 2: ";
cin >> str2;
if (str1 != str2)
cout << "Strings are not equal" << "\n";
else
cout << "Strings are equal" << "\n";
}
Input/Output:
One string is matched against another, and the results are as follows:
Enter String 1:-1
Enter String 2:-1
Strings are equal
In the earlier mentioned example, the comparison operator assesses two strings from their first characters until both strings finish, determining that they are equal.
Conclusion
Ultimately, we've investigated three different approaches for string comparison in C++. One approach involves using strcmp(), which assesses strings by their individual characters. Another way is to use compare() function, offering flexibility for specific comparisons. Also, C++ has relational operators '==' & '!=' signs for simple string checks. Programming professionals can pick any of these methods according to their requirements for making string comparisons more efficient and accurate.