20 Bash Script Examples
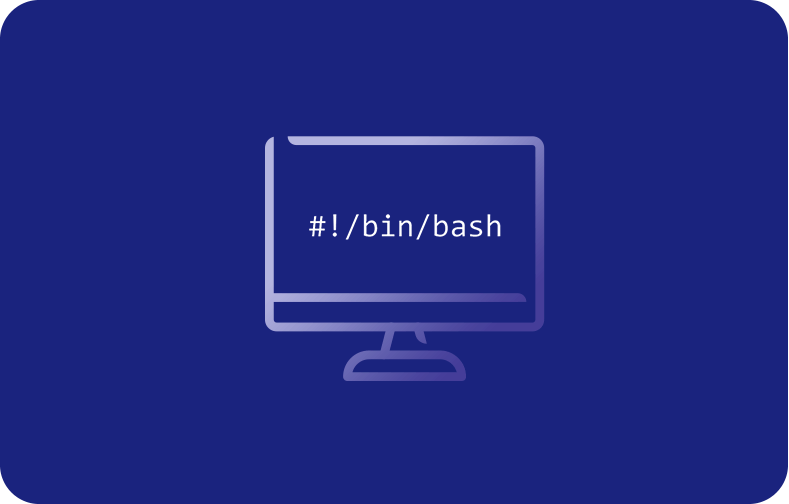
This guide aims to give you an understanding of shell, bash, bash scripting concepts, and syntax, along with some valuable examples. Here, you will learn bash scripting from the ground up and how to automate processes on Linux computers.
We will discuss variables, conditional statements, passing command line arguments to scripts, reading user input, loops and functions, and many more.
-
- What is shell/bash scripting?
- What are the different shell implementations?
- How to create a shell script?
- Tips for writing the script
-
20 Examples to Dive Deeper into Bash Scripting
- Example 01 – Use of variables in scripts
- Example 02 – Inputs and outputs
- Example 03 - Conditional statements with IF
- Example 04 – Case statements
- Example 05 – For loop
- Example 06 – Do while
- Example 07 – Access data from a file
- Example 08 – Check remote server connection
- Example 09 – Delete old files
- Example 10 – Backup file system
- Example 11 – Sleep
- Example 12 – Array
- Example 13 – Bash functions
- Example 14 – Concatenate Strings
- Example 15 – Length of a string
- Example 16 – Find and replace a string
- Example 17 – Check the existence of a file
- Example 18 – Disk status
- Example 19 – System uptime and current date
- Example 20 – Count the number of files
What is shell/bash scripting?
Bash scripting provides a powerful tool for automating tasks on a Linux system. From utilizing the exit status of shell commands to controlling the flow of a script with if-elseif-else statements, bash scripts allow you to harness the power of the command line to perform complex operations.
By using file permissions, you can make sure that only a valid user can access sensitive information. With the bash read command, you can even gather input from a user in the middle of a script.
In addition, the ability to store values as string variables and manipulate string values gives bash scripting a level of versatility comparable to other programming languages.
Whether you're looking to automate simple tasks or write complex scripts, bash scripting is an essential skill for any Linux system administrator.
A file containing a set of executable commands is called a shell script or a bash script. These files have an extension of “.sh”.
What are the different shell implementations?
-
bsh – is known as Bourne shell, which is named after Stephen Bourne.
-
Bash – Bourne Again Shell is an improved version of bash. It is the default shell program for most UNIX-like systems.
Other shell programs such as C shell, Korn shell, etc.
Bash is a shell program. It is a command line bash interpreter and a full-featured programming language for writing shell scripts. So here, we will learn how to write shell scripts using bash syntax. This guide includes twenty (20) bash script examples.
How to create a shell script?
First, you should create a shell script in your terminal. For that, you can use the ‘touch script.sh’ command. You can use any name with the ".sh" extension for the file as all shell script files end with the ".sh" extension.
Tips for writing the script
At the beginning of every script (when writing the script), we should specify which type of shell it should be executed with. This first line of the script is known as the ‘shebang line’. Following are the shebang lines for the Bourne shell, Bourne Again Shell, and C shell.
-
SH - #!/bin/sh
-
BASH - #!/bin/bash
-
ZSH - #!/bin/zsh
The name ‘shebang’ is derived from the "#" and "! " symbols, known as ‘sharp’ and ‘bang’ respectively. Sharp + bang became ‘shebang’ later.
Open and edit the script file
You can use any text editor to open and edit the script file you created. For example, you may use ‘vim script.sh’ or ‘nano script.sh’ to open the file with vim editor or nano editor.
First, we need to write the shebang line, which tells the operating system which shell command program to use to execute the following command lines. Then we may write our first line of script to output a message like this, ‘echo "Hello world!" ’.
You should press ‘s’ to go to ‘INSERT’ mode to start writing on the vim editor. Once your script file is completed, you should press ‘esc’ key and type ‘:’ and ‘w’ and ‘q’ to write changes and quit the vim editor.
Add permissions to your script file
Then, you should look to execute permission on that file. Most probably, you may not have permission to execute the script.sh file, even as the owner of the file.
You can check permissions using the ‘ls –l script.sh’ command. If you do not have execution permission, you can get it by executing the ‘sudo chmod u+x script.sh’ command.
If you run the ‘ls –l script.sh’ command again, you may notice that the executable permissions have been added, and the color of the file name has changed since it is now an executable file.
Script execution
Finally, you can run the ‘./script.sh’ command or ‘bash script.sh’ command to execute the file. Your output will look like the following.
20 Examples to Dive Deeper into Bash Scripting
Since you know the basics, let’s use bash script examples and learn more.
Example 01 – Use of variables in scripts
Our first bash script example is how to use variables in scripts. Bash shell scripts allow us to use variables in different ways. For instance, let’s write our script using two variables, "name" and "place."
Then we can assign a person and place names to the two variables and output them in an echo command statement. You can write a similar script, as follows, and save and run the script on the bash command line.
#!/bin/bash
echo "use of variables in scripts"
echo "==========================="
name="jhon"
place="DC"
echo "$name lives in $place"
You will get an output as shown in the image below.
Example 02 – Inputs and outputs
As of now, you know that we can output streams of strings to the terminal. Additionally, we can get input from the terminal.
In this example, we are going to do that by asking for a name through the terminal. The following code will do the required task. We can use "echo" statements to output strings and add empty lines to the code.
Furthermore, we can use the "read" keyword and a variable to store the given name through the terminal.
#!/bin/bash
echo "Inputs and outputs"
echo "Hi, Im jhon, What is your name ?"
read name
echo "Nice to meet you $name!"
echo "* end of script *"
The following is the output of the script.
Example 03 - Conditional statements with IF
Here, we use a simple if statement to check if a file is available. We can easily understand the syntax of a bash ‘if statement’ as with a normal programming language.
The only difference is that we should use the ‘fi’ keyword to indicate the end of the conditionals. So, let’s try the following bash script.
#!/bin/bash
echo if then else statements
echo
file=abc.txt
if [ -e $file ]
then
echo "Yey, File exists!"
else
echo "file does not exist"
echo "creating the file..".
touch $file
fi
echo "* end of script *"
The output may look similar to the below image.
Example 04 – Case statements
In this bash program, we are outputting some user options and providing identifiers. We are using a command for each option.
Once the user chooses an option, it will execute the relevant command. Here we are using the "uptime" and the "date’ commands for the options.
#!/bin/bash
echo "choose an option:"
echo "1 - system uptime"
echo "2 - date and time"
echo
read choice
case $choice in
1) uptime;;
2) date;;
*) invalid argument
esac
echo
echo "* end of script *"
We should use the ‘esac’ keyword to indicate the end of the case.
The following is the output.
Example 05 – For loop
In this example, we will use a "for loop" to output a certain string a specific number of times. You will be able to grasp the following code example easily.
#!/bin/bash
echo "For loop example"
for i in 1 2 3 4 5
do
echo "$i - Welcome"
done
echo "* end of script *"
Output:
Example 06 – Do while
Using a "do while" loop in bash scripting is simple. Here, ‘-le’ keywords indicate the "less than or equal" sign of a normal programming language.
The following script will run until the variable's value is greater than five.
#!/bin/bash
echo "Do while loop example"
a=1
while [ $a -le 5 ]
do
echo "Hi - Im number $a"
(( a++ ))
done
echo "* End of Script *"
Once you run the script, your output may look the same as the following.
Example 07 – Access data from a file
Usually, we use the "cat" command to read files. We can also use this command inside a script file. First, create a file named abc.txt and it should be empty.
Here, we are trying to read an empty file, "abc.txt," with the command "cat abc.txt."
Then, let’s get the ‘history’ of executed commands and redirect the output to the txt file. Finally, we can use the cat command inside our script and test it out.
#!/bin/bash
cat abc.txt
Output
Example 08 – Check remote server connection
Here, we will ping a destination using an IP address or domain name. In the script, first we ask the user to enter an IP address (or else you can provide it as google.com).
Then read the input and use it with the "ping" command. After that, we can get the ping command output and display it.
We will output a message based on the status of the ping, indicating whether it’s successful or not. The command ‘ping –c2 $ip’ instructs to ping only the given destination twice.
As you already know, exit states 0 indicates success in the ping command. If it is "0," we display a "success" message; otherwise, we display a "try again" message.
#!/bin/bash
echo "Enter an ip address to ping test :"
read ip
echo "you gave - $ip"
echo "pinging > +++++++++++++++++++++++++++++++"
ping -c2 $ip
if [ $? -eq 0 ]
then
echo
echo "=============================================="
echo "Ping success!"
else
echo "----------------------------------------------"
echo "Ping not success!, try again.."
fi
echo
echo "* end of script *"
Below is the output.
Example 09 – Delete old files
We can write a script to remove old files. We can do this by determining how old a script is and deleting it from a specific location.
Let’s say we are going to delete files older than 90 days. If you do not have older files, you can create them with the command, ‘touch -d "Fri, 2 September 2022 12:30:00" file1’.
The file is called file1 and was created on September 2nd, 2022. Then we can use the command ‘find /home/test/scripts -mtime +90 -exec rm {} \;’ to remove those files.
Additionally, you may use the command ‘find /home/test/scripts -mtime +90 -exec ls -l {} \;’ to find and list them if you want.
#!/bin/bash
find /home/test/scripts/ -mtime +90 -exec rm {} \;
Output:
Example 10 – Backup file system
In the following script, we will create a backup of the ‘backup’ directory using tar. Then we use gzip to compress that backup file, "scripts-backup.tar" to ‘scripts-backup.tar.gz’. Finally, output a message as ‘process finished’.
#!/bin/bash
tar cvf /home/test/scripts/scripts-backup.tar /home/test/scripts/backup
gzip scripts-backup.tar
echo "process finished!"
The following figures show how the directory looks before and after running the script file.
Before:
After:
Example 11 – Sleep
Here we can use the sleep command to put the system to sleep. We can set a sleep time and check how it is working.
The sleep command pauses the process and puts the system to sleep. Let’s try it out now. Here, we use the sleep command with a ‘for loop’, giving you a good night message every 20 seconds.
#!/bin/bash
echo "For loop with sleep timer"
for i in 1 2 3 4 5
do
echo "Im going to sleep - day $i" && sleep 20
done
echo "* end of script *"
Output
Example 12 – Array
A bash array is a data structure for storing information like an index. It is useful when storing data and reading it in different ways later.
These arrays are good for storing different elements, such as strings and numbers. Here we are utilizing an array that contains students and reading them one by one using a "for a loop."
#!/bin/bash
students=(jessica jhon dwain sian rose mary jake123 sam303)
echo "Read data from an array"
for i in "${students[@]}"
do
echo "Student name : $i"
done
echo "* end of script *"
Output
Example 13 – Bash functions
We can write functions in bash scripts as in a normal programming language and use them inside script files whenever we want.
Here, we use an array of guests and welcome each with a greeting message. We can call this bash function by name once we use it inside a script.
#!/bin/bash
welcome () {
guests=(jessica jhon dwain sian rose mary jake123 sam303)
echo "Write an array inside a Function"
for i in "${guests[@]}"
do
echo "Welcome $i as a guest!"
done
}
welcome
Output
Example 14 – Concatenate Strings
We can append a string to another string, known as concatenating string. We use a fourth variable to concatenate three variables that store strings.
Finally, we can output the appended string.
#!/bin/bash
beginning="Jhon "
middle="was born in"
end=" USA"
apendedvalue="$beginning$middle$end"
echo "$apendedvalue"
Output
Example 15 – Length of a string
This is a straightforward example where we can write the script file and get the output. Here, we assign a sentence to a variable and count its length.
#!/bin/bash
echo "Below is the sentence"
echo "+++++++++++++++++++++++"
echo
echo "how long is this sentence?"
echo
sentence="how long is this sentence?"
length=${#sentence}
echo "Length of the sentence is :- $length"
Output
Example 16 – Find and replace a string
This bash script demonstrates the ability to find and replace a string in a file using shell scripting. The script allows for simple manipulation of a file, allowing users to find and replace strings as needed.
By running the script, a new bash file is created which replaces the specified string with a new one, ensuring that the information in the file is up-to-date and accurate.
This makes it a useful tool for users who need to modify files, especially those who are managing a large number of files or a running process.
The script is written in bash, and can be run as a standalone bash file or incorporated into a larger script.
The use of shell scripting in this example makes it easy for users to quickly and easily manipulate files, allowing them to find and replace strings with ease.
Whether working with a single file or managing a complex system, this script demonstrates the power and versatility of bash scripting.
#!/bin/bash
echo "please enter a string"
read input
echo "Enter the word you need to replace -"
read rep
echo "enter the replacement"
read replacement
echo
echo "Replaced sentence - ${input/$rep/"$replacement"}"
Below is the output.
Example 17 – Check the existence of a file
The following script demonstrates the ability to check the existence of a file on a system using the command line. The script takes user input in the form of a string value, which represents the file name that is to be checked.
By using the "test" command, the script can quickly and easily determine whether the file exists or not. The script outputs a message indicating the result of the check, and can be run as a standalone sh file or included in a larger bash script.
This simple but powerful bash file demonstrates the ease with which users can manipulate files and retrieve information from the command line using bash scripts.
Compared to an other programming language, bash scripts allow for a much more streamlined and efficient process, as users can quickly write, test, and run scripts without having to write large amounts of code.
Additionally, the use of the "test" command ensures that the script works seamlessly across different systems and platforms, making it an excellent tool for any bash user.
#!/bin/bash
file=file2.txt
echo "whats inside this directory ?"
ls -l
echo
if [ -f "$file" ]
then
echo "$file exists"
else
echo "$file does not exist - creating the $file"
touch file2.txt
fi
echo "Whats inside this directory ?"
ls -l
Output
Example 18 – Disk status
This bash script demonstrates the use of the "df -h" command to retrieve disk space details on a system. The script uses the command line and the bash command to quickly and easily gather information about the disk status.
By running the script with the "sudo" command, it is possible to retrieve information about the disk space and usage even for running processes and files that may be owned by other users. The script creates a file named "disk_status.txt" to store the information gathered, ensuring that the information is easily accessible and retrievable.
This bash file demonstrates the versatility and power of bash scripting, allowing users to gather information about the system without having to manually input commands or navigate through the file system. With just a single command, this script provides a quick and convenient way to retrieve information about the disk status of a system.
#!/bin/bash
df -h
Output
Example 19 – System uptime and current date
This bash script uses the "uptime" and "date" commands to get information about the system uptime and current date. The script starts by creating a string variable named "today" and assigning the result of the "date" command to it.
The script then outputs a message which includes the string value of "today" with the text "SYSTEM UPTIME info -".
This simple bash script demonstrates the power of the command line and the versatility of bash scripting. By using the wait command and string variables, this script allows a user to quickly and easily get information about the system's uptime and current date without having to manually type the commands or navigate through the file system.
With the ability to create a file named "system_uptime.txt", a user can even save this information for later use, ensuring that an invalid user doesn't have access to it.
#!/bin/bash
today=`date`
echo "SYSTEM UPTIME info - $today"
echo "+++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++"
uptime
Output
Example 20 – Count the number of files
The following bash script demonstrates the ability to count the number of files within a directory. This is an important task for any Linux user, as it allows you to monitor the contents of a directory and ensure that only a valid user has access to the files within it.
This script first uses the ls -l command to list the files in the directory, including their permissions and the process ID of the file owner. It then prompts the user to answer "how many files are here?" and uses the ls -l * | wc -l command to count the number of files, including any text file.
#!/bin/bash
ls -l
echo "how many files are here?"
ls -l * | wc -l
Output
That’s it!
If you have routine tasks on your Linux computer, doing the same thing over and over will be tedious and time-consuming. This is where bash scripting comes in handy. You can write all those terminal commands in one script file and execute them whenever possible.
There is no need to type every command from scratch. Isn’t it a lifesaver? Moreover, you can easily share script files among a team. It will save you and your colleagues a lot of time and effort.
It will also avoid repetitive work, let you share instructions, help in logical and bulk operations, and make it easy to keep configuration histories for tasks, projects, or devices. So hopefully, we assume you have gained good knowledge about automating your tasks with our bash scripting tutorial.
Frequently Asked Questions
What is the "wait" command in Bash programming?
The "wait" command waits for a specified process to complete before continuing the execution of the script.
How to write a multi line comment in Bash Scripting?
A multi line comment can be written in Bash Scripting by enclosing the text between "#" symbols, with one symbol at the beginning and end of each line of the comment.
How to read command line arguments in Bash Scripting?
Command line arguments can be read in Bash Scripting by using the special variables "$1", "$2", "$3", etc. to access each argument in order. If you use two command line arguments, $2 will be the second argument.
How to perform conditional tests in Bash Scripting?
Conditional tests can be performed in Bash Scripting using the "if" statement, where the statement inside the "if" block is executed only if the specified condition is met.
How can I check for a existing file in a bash script?
Use the if [ -f "file.txt" ]; then command to check if a file named "file.txt" exists.
How can I read user input in a bash script?
Use the read command to read user input, e.g. read -p "Enter a value: " value.
How can I check if a directory exists in a bash script?
Use the if [ -d "dir" ]; then command to check if a directory named "dir" exists.
How do I write scripts to run in the current directory?
Use ./script.sh to run a script named "script.sh" in the current directory.